Code Snippets
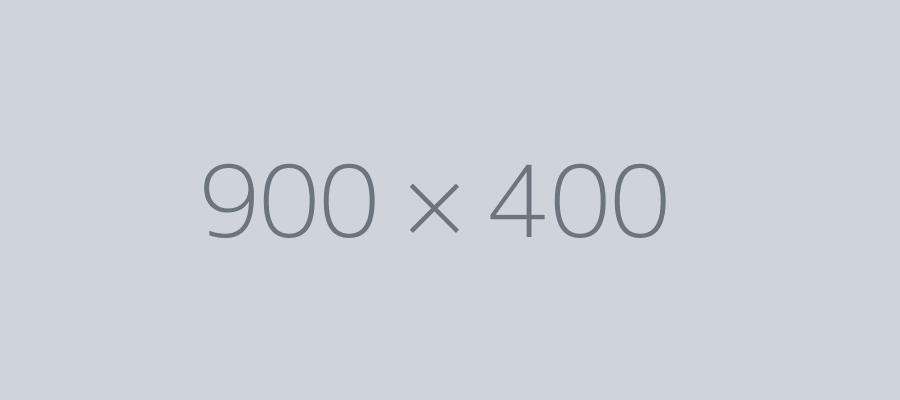
When you start your journey "under the hood", you will find that you are using much of the same code throughout many of your projects. Finding these "golden nuggets" of code can take years if you are trying to figure this out on your own. Luckily for you, I have compiled a collection of the most common code snippets used through this site to fully integrate every aspect of the site into one cohesive unit.
Article Custom Fields
Including custom fields anywhere in your template that uses the 'Article Object' programmatically can be accomplished using the following steps:
-
- Add the following line to the top of the php file you want to the custom field to display on:
use Joomla\Component\Fields\Administrator\Helper\FieldsHelper;
- Depending on where the article object is being called (if in module or category page it would be inside a 'foreach' array, if in an article page, anywhere in the top php code would work):
$jcFields = FieldsHelper::getFields('com_content.article', $article, true);
foreach($jcFields as $jcfield)
{
$jcFields[$jcfield->name] = $jcfield;
} - Add the custom fields you wish to include after the code in Step 2:
$mycustomfield = $jcFields['mycustomfield-alias']->rawvalue;
- Add the following line to the top of the php file you want to the custom field to display on:
Breaking down the following line from Step 2:$jcFields = FieldsHelper::getFields('com_content.article', $article, true);
- 'com_content.article': This tells the code to look for custom fields related to the 'Article Object'
- '$article': is the 'Article Object' that the custom fields are going to be pulled from
- 'true':indicates whether to display all the field data or (false) just the raw data - changing to false will shave a little time off the page load (especially if you are using a lot of custom fields)
Breaking down the following line from Step 3:$mycustomfield = $jcFields['mycustomfield-alias']->rawvalue;
- '$mycustomfield': This can be whatever unique name you prefer to use to name your custom field variable (as long as it starts with '$')
- 'mycustomfield-alias': Alias name of the article custom field.
- 'rawvalue':indicates we are using the "raw" value of the custom field as opposed to the
$mycustomfield = $jcFields['mycustomfield-alias']->value;
That displays the default layout display of the custom field.
Finding the 'Object'
An 'Object' can be best described as a collection of data for a specific item. In the code snippets about, we are trying to find the 'Article Object'. This can vary depending on the page/module type.
Article Object Cheat Sheet
- Article Category Page - $article (inside the 'foreach' statement)
$jcFields = FieldsHelper::getFields('com_content.article', $article, true);
- Single Article Page - $this->item
$jcFields = FieldsHelper::getFields('com_content.article', $this->item, true);
- Article Category Module - $item (inside the 'foreach' statement)
$jcFields = FieldsHelper::getFields('com_content.article', $item, true);
Article Custom Field Example
The following example will add add article custom fields to an 'Articles - Category' module using a layout override:
- Create a 'Text Type' custom field titled 'Tester 1' with the alias 'tester-1' and add some text to the field for the selected article that will display in the module.
- Create a new layout override for the module:
- 'System > Site Templates > [Your Template] > Create Overrides'
- Under 'Modules' click 'mod_articles_category'
- Select the 'Editor' tab on the same screen then look for the html folder
- Article Category Page - $article (inside the 'foreach' statement)
$jcFields = FieldsHelper::getFields('com_content.article', $article, true);